The `cmd /c` command in PowerShell allows you to execute a command specified as a string, and then terminates after the command has been completed.
Here’s a code snippet demonstrating its use:
cmd /c echo Hello from CMD
Understanding PowerShell
What is PowerShell?
PowerShell is an advanced task automation framework that combines a command-line shell with scripting capabilities. It is particularly powerful for system administration and is designed to help administrators automate the management of operating systems and applications. Built on the .NET framework, PowerShell utilizes cmdlets, which are specialized .NET classes, to perform various functions, providing a robust environment for executing administrative tasks.
PowerShell vs CMD
While both PowerShell and the Command Prompt (CMD) are command-line interfaces, there are fundamental differences in functionality and purpose:
- PowerShell is built for automation and configuration management, supporting complex scripting and the management of remote systems.
- Command Prompt is a simpler command-line application that executes commands without the extensive features and flexibility of PowerShell.
When deciding between the two, choose PowerShell for its rich set of features, especially when tasked with automating repetitive administrative tasks.
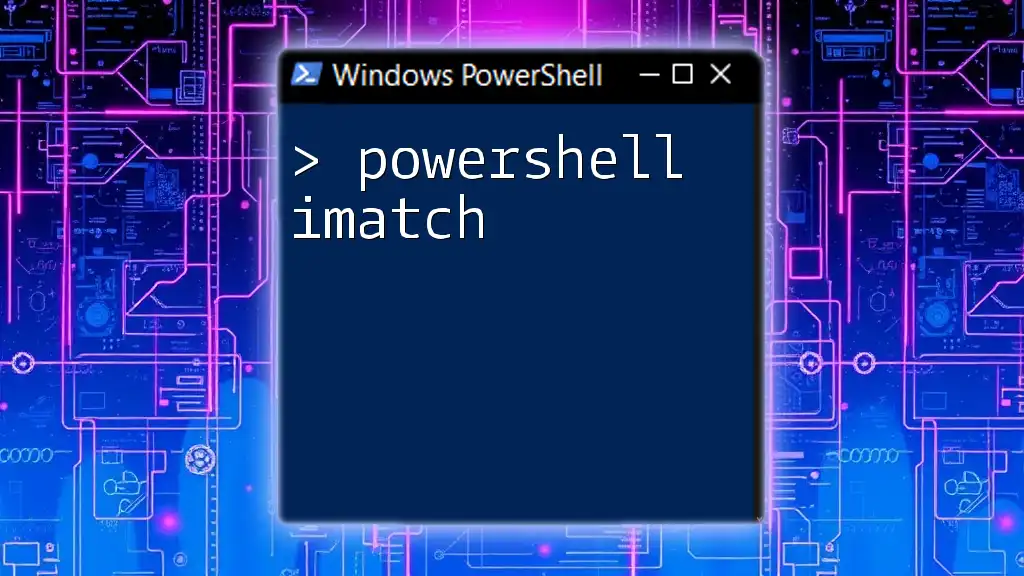
What is the `/c` Parameter in CMD?
Basic Definition
The `/c` switch in the Command Prompt (CMD) is a command-line parameter that tells CMD to execute a command and then terminate. This means that any command you specify will run to completion before the CMD window closes.
How `/c` Translates to PowerShell
In PowerShell, utilizing `cmd /c` allows you to execute a CMD command directly from the PowerShell environment. This is particularly handy for leveraging existing CMD tools or scripts without having to rewrite them for PowerShell.
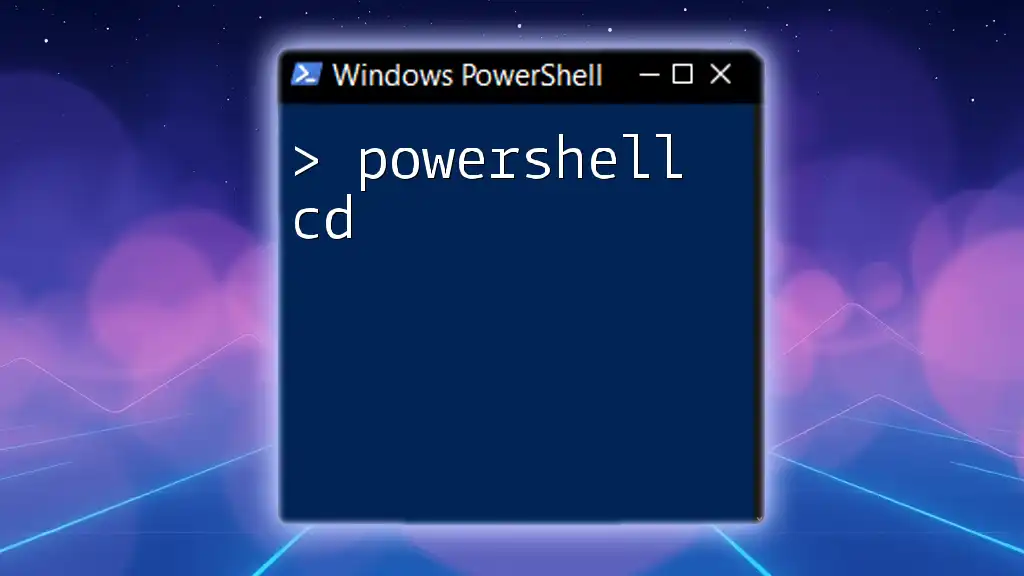
Using `cmd /c` in PowerShell
Syntax and Structure
The basic syntax for invoking a CMD command from PowerShell using the `/c` parameter is as follows:
cmd /c "your-command-here"
Examples of `cmd /c` Usage
Example 1: Running a Simple Command
To demonstrate the simplicity of running CMD commands via PowerShell, consider the following example:
cmd /c "echo Hello World"
Explanation: This command calls CMD to execute the `echo` command, which outputs "Hello World" to the console. It's a straightforward way to see how seamlessly PowerShell can run CMD commands.
Example 2: Executing Batch Files
Another powerful use case is executing batch files from PowerShell. For instance, if you have a batch file located at `C:\Path\To\YourScript.bat`, you can run it in the following way:
cmd /c "C:\Path\To\YourScript.bat"
Explanation: This example uses the `/c` parameter to read and execute a batch file directly from PowerShell, allowing you to integrate legacy scripts into modern workflows.
Passing Parameters with `cmd /c`
When you need to pass parameters to a command, `cmd /c` can handle that as well. For example, let's see how to list the files in a directory:
cmd /c "dir C:\Users\Public"
Explanation: This command retrieves a directory listing for `C:\Users\Public`, showcasing how you can use CMD's parameters within a PowerShell script.
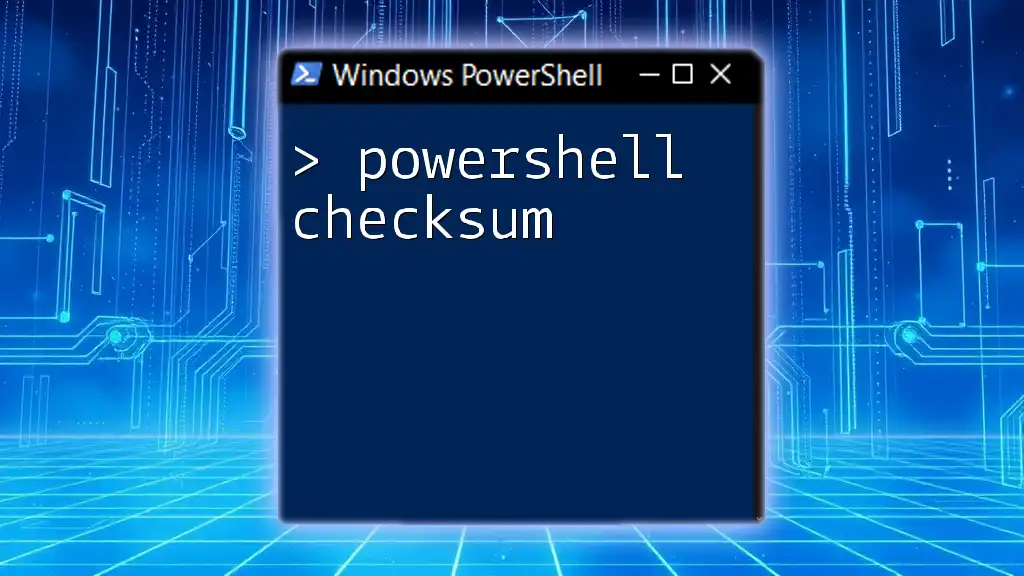
Advantages of Using `cmd /c` in PowerShell
Flexibility in Command Execution
One of the primary benefits of using `cmd /c` in PowerShell is the flexibility it provides. By allowing you to execute native CMD commands within the robust environment of PowerShell, you can tackle a broader range of tasks without having to rewrite script logic.
Legacy Support
Using CMD commands ensures compatibility with legacy applications. Many organizations still rely on older scripts that are written in batch, and `cmd /c` allows these processes to function within a PowerShell environment.
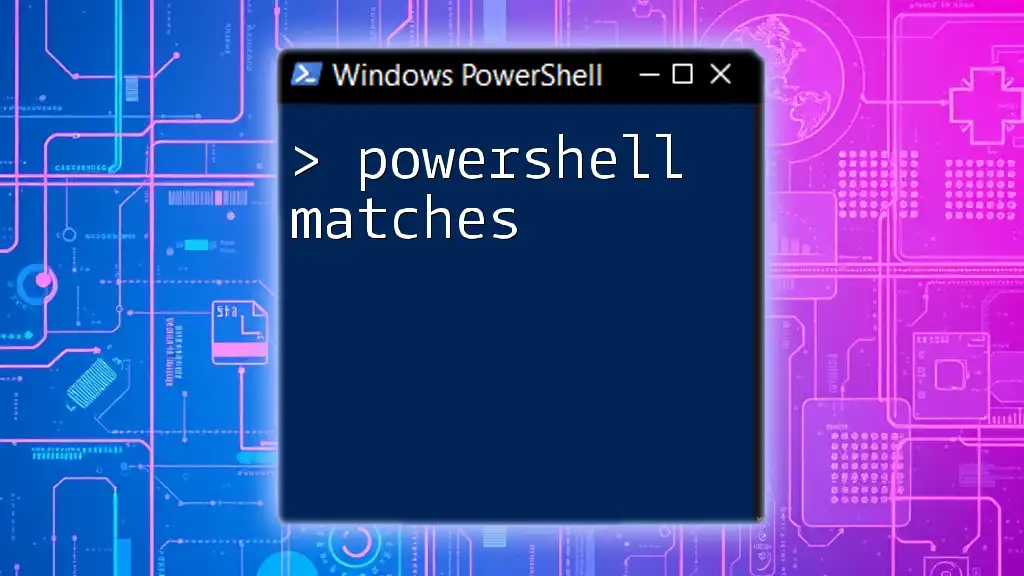
Common Pitfalls and Troubleshooting
Error Handling
When executing commands through `cmd /c`, you might encounter errors if the command or script path is incorrect. Using `try` and `catch` blocks in PowerShell can help handle errors gracefully. For example:
try {
cmd /c "your-command"
} catch {
Write-Host "An error occurred: $_"
}
Explanation: This snippet shows how to catch errors when running CMD commands, providing a fallback to make your scripts more stable.
Misunderstanding Context Switching
When using `cmd /c`, it's essential to understand that you're switching from the PowerShell context to the CMD context. Be aware that not all PowerShell features and syntax are compatible in CMD, which may lead to confusion among users.
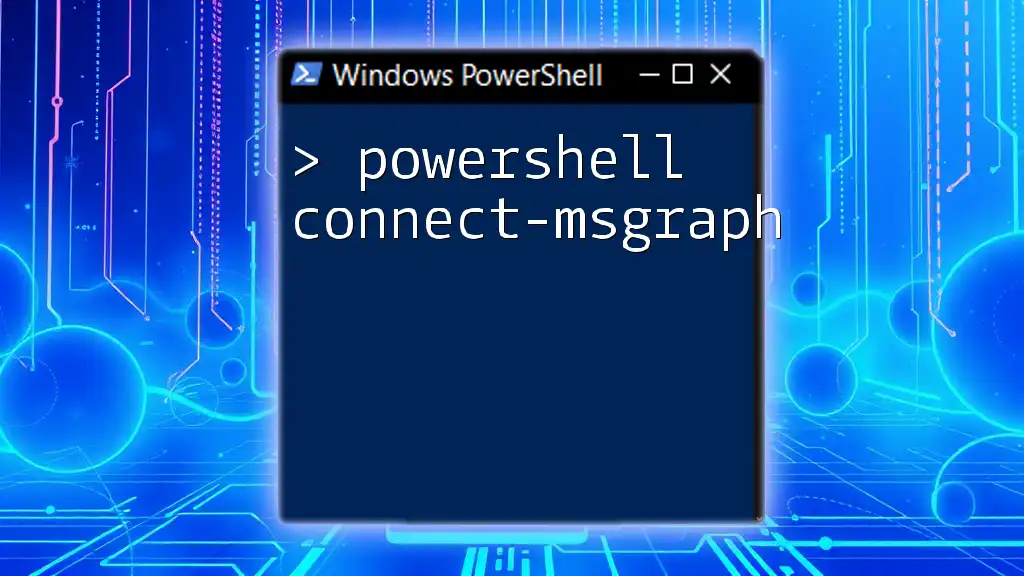
Best Practices When Using `cmd /c` in PowerShell
Limitations to Keep in Mind
While using `cmd /c` is beneficial, there are limitations, such as:
- Lack of PowerShell's advanced features like object-oriented output.
- Potential constraints with error handling as CMD has a different way of reporting errors than PowerShell.
Combining PowerShell Script Features with CMD
To build effective hybrid scripts, mix PowerShell's object-oriented capabilities with CMD's straightforward command execution. This combination allows for more readable, efficient, and powerful scripts.
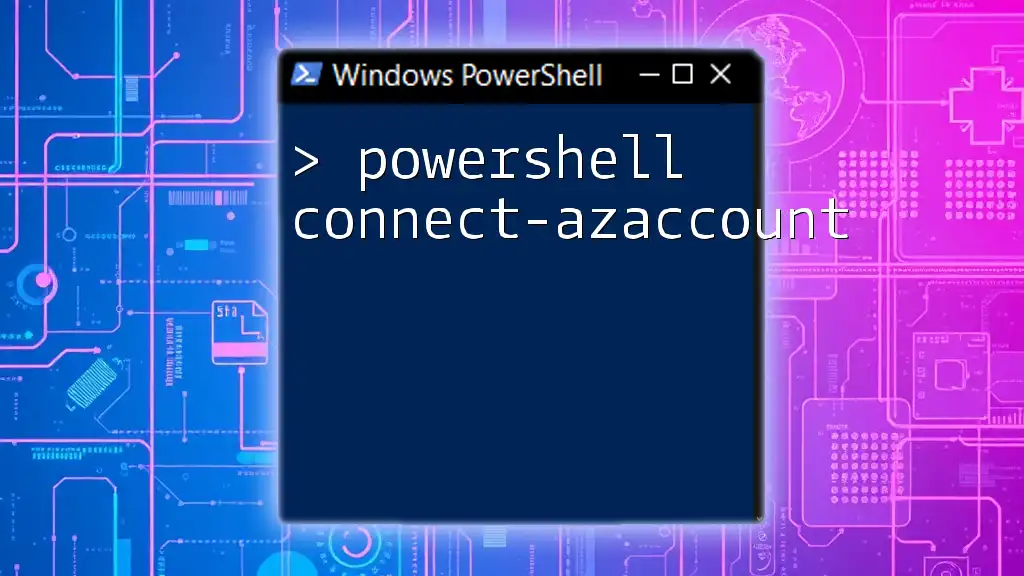
Conclusion
In conclusion, understanding how to properly use `PowerShell cmd /c` opens up a wealth of possibilities for automation and scripting. By leveraging the strengths of both PowerShell and CMD, you can create dynamic solutions that efficiently perform system tasks. Experiment with the examples provided, and consider how you can apply this knowledge to streamline your workflow.
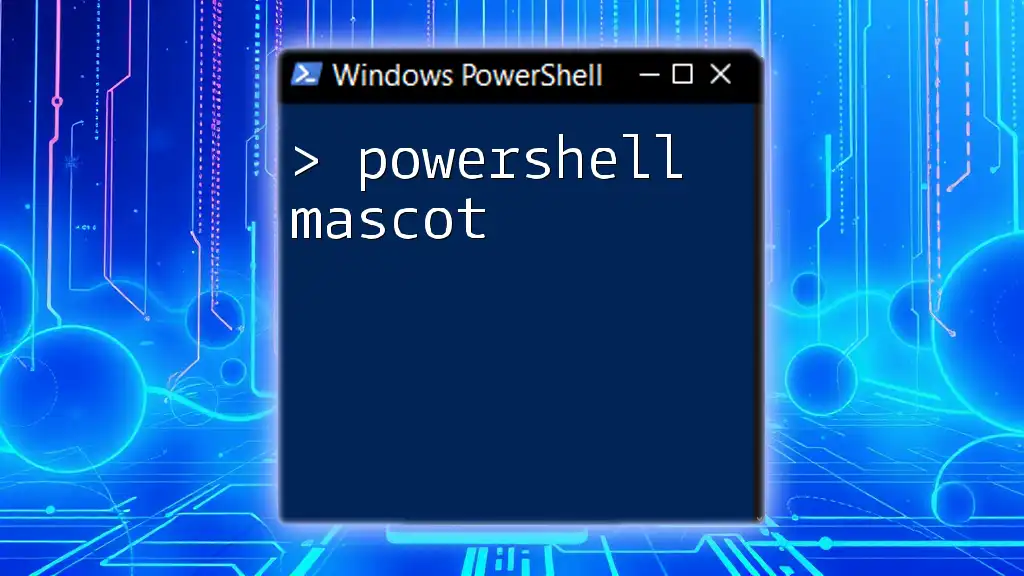
Additional Resources
Recommended Reading
For further exploration of PowerShell and CMD, consider diving into books and online resources that focus on task automation and scripting techniques.
Community Support
Don’t hesitate to seek guidance from online forums and communities where you can pose questions, share experiences, and learn from others exploring the depths of PowerShell.
FAQ Section
In this section, you can anticipate common queries regarding the usage and limitations of `cmd /c` within PowerShell, providing more clarity to your audience.
By implementing these practices, you can efficiently harness the power of PowerShell along with the simplicity of CMD to maximize your productivity.