In PowerShell, the `New-Item` cmdlet is used to create a new folder within a specified path.
New-Item -Path "C:\ExampleFolder" -ItemType Directory
Understanding PowerShell and Its Purpose
What is PowerShell?
PowerShell is a powerful task automation and configuration management framework from Microsoft, comprised of a command-line shell and a scripting language. It is built on the .NET framework and is designed specifically for system administrators and developers to automate the management of operating systems and the applications running on them. With its extensive set of cmdlets, scripts, and flexible syntax, PowerShell is equipped to handle a wide range of tasks—from simple file operations to complex system management tasks.
Importance of Folder Management
Effective file and folder management is crucial for maintaining organized data, especially in both personal and professional settings. Properly structured folders help in quick accessibility, efficient backup processes, and overall better system performance. By using PowerShell to automate folder creation, users can save substantial time and avoid errors related to manual folder setup, enhancing their productivity.
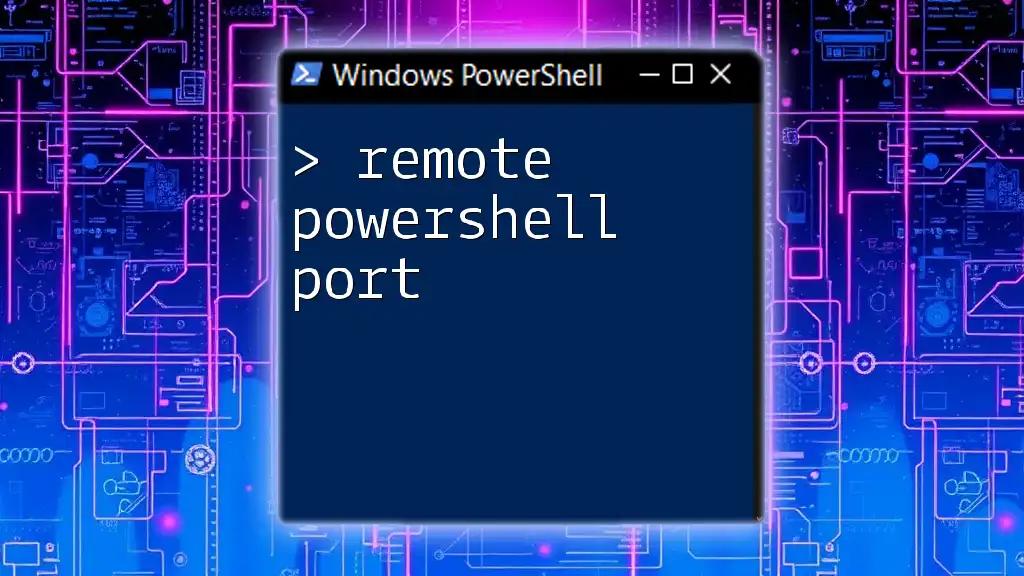
Getting Started with New-Item
The New-Item Command
The `New-Item` cmdlet in PowerShell is an essential tool that allows users to create new items—be it files, folders, or even registry keys. When it comes to creating folders, `New-Item` simplifies the process significantly, making it quick and straightforward to establish new directory structures.
Syntax Breakdown
The basic syntax of the `New-Item` command is as follows:
New-Item -Path <string> -Name <string> -ItemType <string>
- -Path: Specifies the location where you want to create the folder.
- -Name: Defines the name of the new folder.
- -ItemType: Indicates the type of item to create, in this case, a directory (folder).
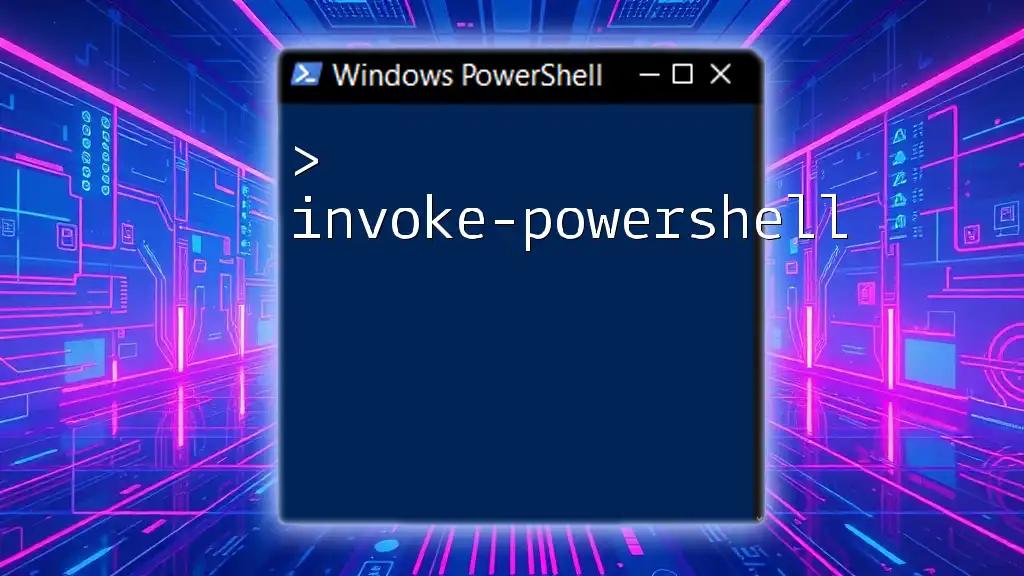
Creating a Basic Folder with New-Item
Example of a Simple Folder Creation
Creating a simple folder using `New-Item` is as easy as running the following command:
New-Item -Path "C:\ExampleFolder" -ItemType Directory
In this example, the cmdlet tries to create a new folder named "ExampleFolder" at the root of the C drive. If the command passes without errors, a folder will appear at the specified location.
Exploring Folder Creation with Path and Name
To create a folder with a unique name in a specified path, you can combine the `-Path` and `-Name` parameters:
New-Item -Path "C:\Users\YourUsername\Documents" -Name "MyNewFolder" -ItemType Directory
Here, "MyNewFolder" will be created inside the "Documents" directory of the specified user. This separation allows for clearer identification and organization of multiple folders.
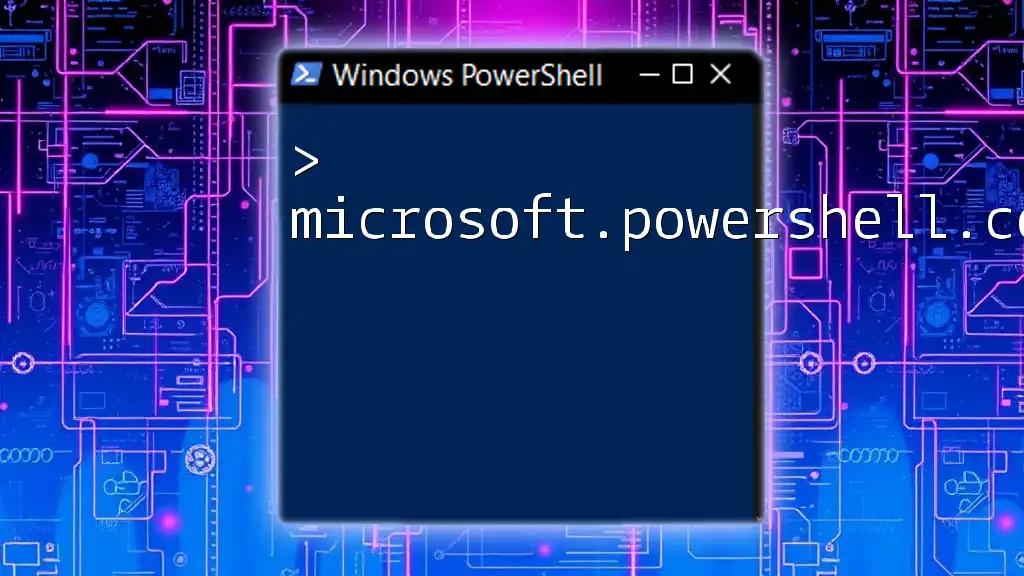
Advanced Usage Scenarios
Creating Nested Folders
One of the powerful features of `New-Item` is its ability to create nested folders in a single command, allowing for better organization:
New-Item -Path "C:\ParentFolder\ChildFolder" -ItemType Directory
This command creates a structure where "ChildFolder" is a subfolder within "ParentFolder." Utilizing nested folders can help keep files organized in a systematic manner.
Checking for Existing Folders
Using If Condition
To ensure you don’t encounter errors when trying to create a folder that already exists, you can use an if condition. This practice is crucial for smooth operation, especially in scripts:
if (!(Test-Path "C:\ExampleFolder")) {
New-Item -Path "C:\ExampleFolder" -ItemType Directory
} else {
Write-Host "Folder already exists."
}
This script first checks if "ExampleFolder" exists. If it does not, it creates the folder; otherwise, it prints a message to the console, helping to avoid duplicate creation attempts.
Creating Folders with Dynamic Names
Using variables for folder names can add flexibility to your scripts. For example, you may want to include a timestamp in the folder names to avoid conflicts:
$folderName = "DynamicFolder_" + (Get-Date -Format "yyyyMMdd")
New-Item -Path "C:\Example\" -Name $folderName -ItemType Directory
In this scenario, the new folder created will contain the current date in its name, making it easy to identify when it was created.
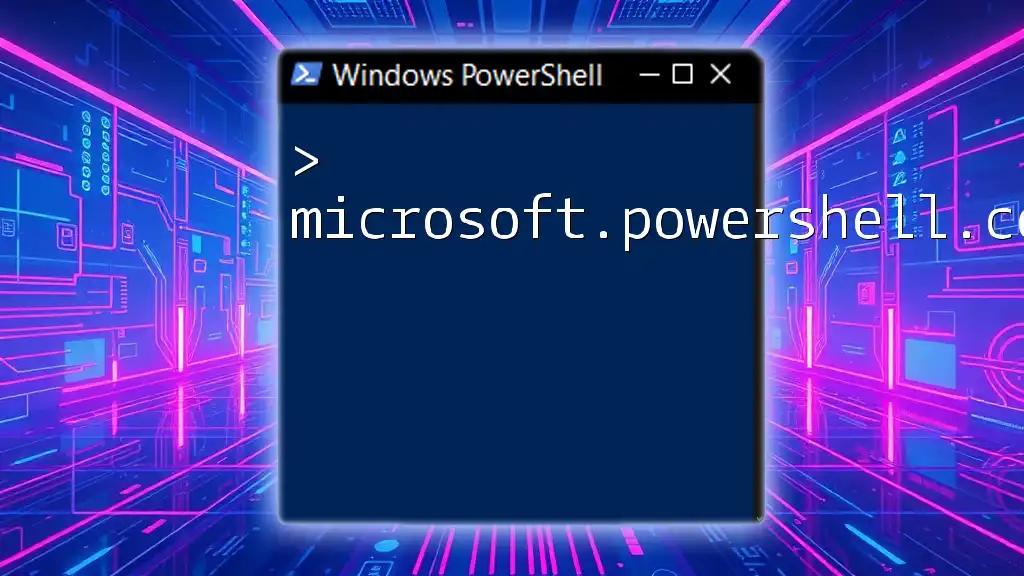
Error Handling in Folder Creation
Common Errors Encountered
While creating folders with `New-Item`, users may encounter various errors, such as permission issues or specifying an invalid path. It's essential to know how to handle these errors gracefully.
Implementing Try-Catch for Error Management
You can use a try-catch block to manage potential errors effectively. This allows you to catch exceptions and take appropriate actions if an error occurs during folder creation:
try {
New-Item -Path "C:\RestrictedFolder" -ItemType Directory
} catch {
Write-Host "An error occurred: $_"
}
In this code snippet, if the folder creation fails due to any reason (like lack of permissions), the catch block will execute, informing the user of the error rather than halting the script.
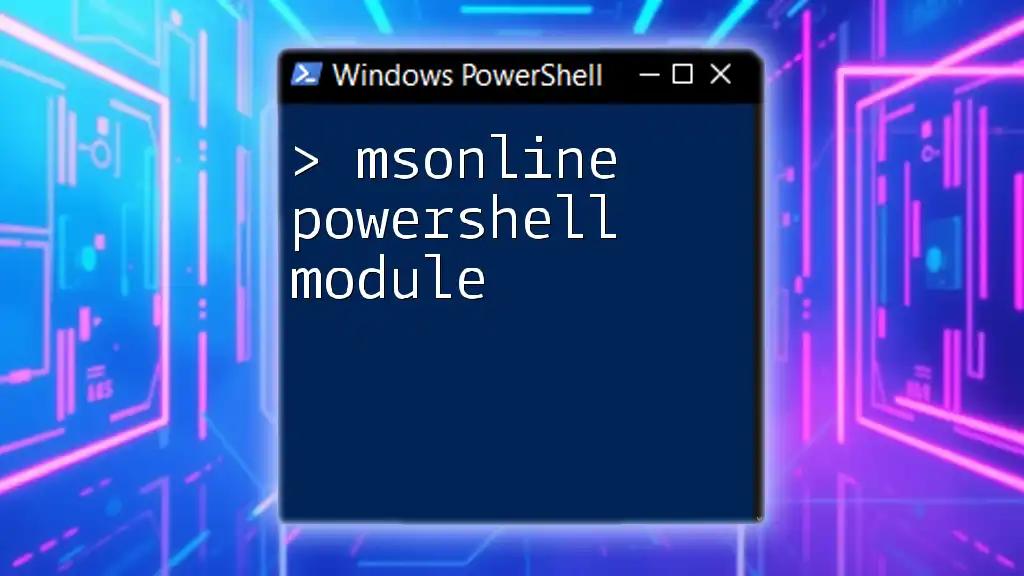
Conclusion
Recap of New-Item Usage
In this comprehensive guide, we’ve explored how to leverage the `New-Item` cmdlet in PowerShell to create folders. We discussed basic syntax, advanced usage scenarios, and best practices for error handling, equipping you with the necessary skills to manage folders effectively through PowerShell.
Encouragement for Continued Learning
Experimenting with PowerShell will broaden your automation skills and allow you to explore its extensive capabilities further. Using the `New-Item` cmdlet is just the beginning—there's a wealth of features waiting for you to uncover.

Additional Resources
Links to PowerShell Documentation
For an in-depth understanding of PowerShell and its cmdlets, visit the official Microsoft PowerShell documentation. They provide exhaustive details and examples that will enhance your learning process.
Suggested Exercises
To solidify your understanding of folder creation using `New-Item`, try creating various folder structures. Challenge yourself with dynamic naming and error handling scenarios to ensure you are well-versed in practical applications.
Join the Community
Don’t hesitate to engage with fellow PowerShell users in forums or community groups. Learning from shared experiences and expertise can provide invaluable insights into mastering PowerShell scripting and automation.