The SCCM PowerShell detection method allows administrators to use PowerShell scripts to determine if an application is installed on a system by evaluating specific criteria.
# Example SCCM Detection Method
$installedApp = Get-WmiObject -Class Win32_Product | Where-Object { $_.Name -eq "YourApplicationName" }
if ($installedApp) {
Write-Host 'Application is installed.'
} else {
Write-Host 'Application is not installed.'
}
Understanding Detection Methods
What is a Detection Method?
Detection methods in SCCM (System Center Configuration Manager) are crucial for ensuring that applications are correctly deployed and managed. These methods determine whether an application is installed on a target machine before executing certain actions, such as updating or reinstalling software.
Detection methods help in maintaining system integrity by preventing unnecessary installations and ensuring that deployments only happen when required.
Types of Detection Methods
There are several types of detection methods you can implement in SCCM, each serving its specific context:
- Script-based detection: Involves the execution of scripts to verify the existence or status of an application.
- Registry-based detection: Uses Windows registry entries to determine whether an application is installed.
- File existence detection: Checks for specific files on the file system that denote successful application installation.
- Windows Management Instrumentation (WMI): Queries the system for installed applications through WMI classes.
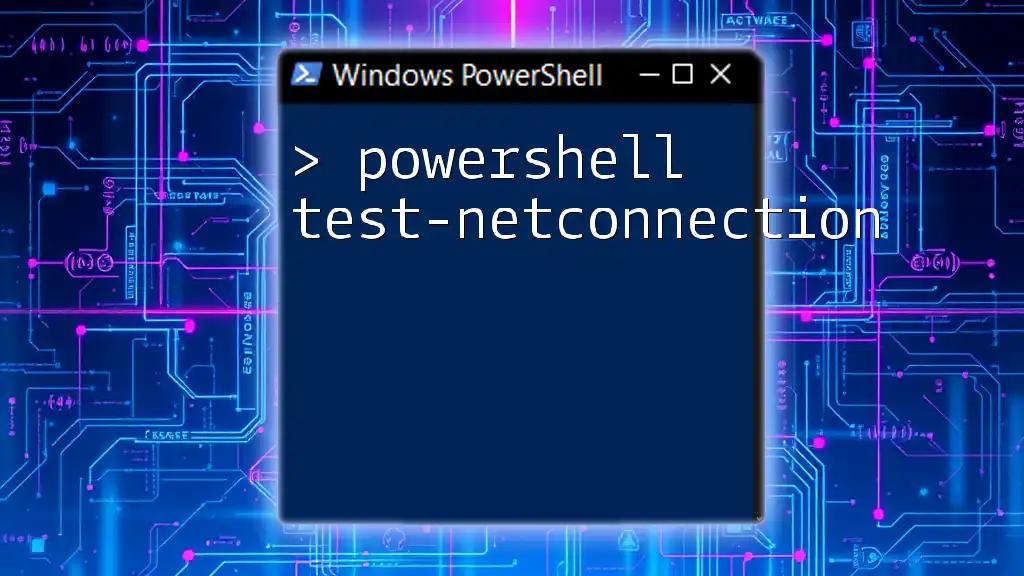
Leveraging PowerShell for Detection Methods
Why Use PowerShell?
PowerShell serves as a powerful scripting language and shell that allows IT professionals to automate tasks and manage configurations efficiently. Its integration with SCCM provides administrators with a robust tool for creating custom detection methods.
With PowerShell, you can streamline your workflow, utilize complex logic in detection scripts, and enhance your overall management capabilities through automation.
Getting Started with PowerShell in SCCM
Prerequisites
Before you dive into using PowerShell with SCCM, ensure that your environment is set up appropriately. You should have access to your SCCM site and be familiar with the SCCM console.
- Install the SCCM PowerShell module: This module allows you to run cmdlets designed for SCCM management.
- Verify your access rights: Ensure you have administrative privileges to execute SCCM commands.
Basic PowerShell Command Structure
PowerShell commands, known as cmdlets, are constructed using a standard verb-noun format. Here’s a basic example to get you started:
Get-Process
In this command, `Get` is the verb, and `Process` is the noun. This command retrieves a list of active processes on your system.
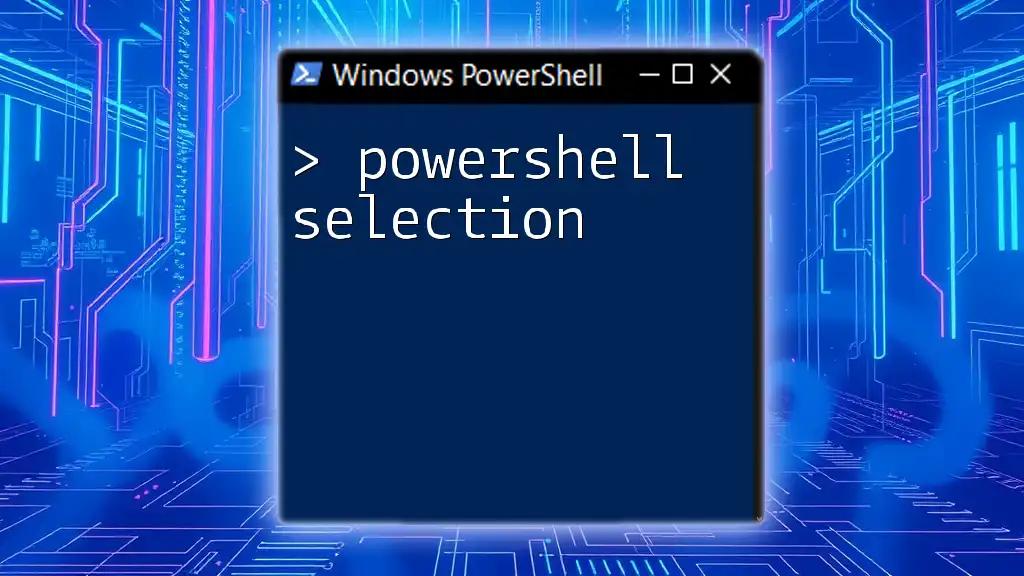
Creating a Detection Method using PowerShell
Script-based Detection
Writing Your Detection Script
Creating a script-based detection method can be done using native PowerShell commands. Below is an example detection script:
$AppName = "Your App Name"
if (Get-Command $AppName -ErrorAction SilentlyContinue) {
Write-Output "$AppName is installed"
exit 0
} else {
Write-Output "$AppName is not installed"
exit 1
}
In this script, we attempt to get the command for the application. If successful, it indicates that the application is installed, and the script exits with a status of `0`. If not, it returns a status of `1`, signifying the application isn't found.
Registry-based Detection
Accessing Registry Keys
Registry-based detection checks for a specific key in the Windows registry to verify installation. Here’s how you can do that:
$RegPath = "HKLM:\Software\YourSoftware"
$RegValue = "Installed"
if ((Get-ItemProperty -Path $RegPath).$RegValue -eq 1) {
Write-Output "Software is installed."
exit 0
} else {
Write-Output "Software is not installed."
exit 1
}
In this example, the script retrieves the specified registry key and checks the value associated with it. If it equals `1`, the software is considered installed.
File Existence Detection
Verifying Installed Files
Another common detection method is checking if specific files exist on the computer:
$FilePath = "C:\Program Files\YourSoftware\yourfile.exe"
if (Test-Path $FilePath) {
Write-Output "File exists."
exit 0
} else {
Write-Output "File does not exist."
exit 1
}
This script uses the `Test-Path` cmdlet to verify whether the specified file exists. A successful check leads to a positive confirmation of application installation.
WMI-based Detection
Querying WMI for Installed Applications
WMI-based detection queries the system for installed applications in a more thorough way:
$WmiQuery = "SELECT * FROM Win32_Product WHERE Name = 'Your App Name'"
if (Get-WmiObject -Query $WmiQuery) {
Write-Output "Application is installed."
exit 0
} else {
Write-Output "Application is not installed."
exit 1
}
This script queries the `Win32_Product` class for the specified application name. If found, it confirms installation.

Best Practices for Using PowerShell Detection Methods in SCCM
Testing Your Detection Methods
Always test your PowerShell scripts in a safe environment before deploying them widely. You can run scripts on a local machine to confirm their functionality. This hesitance minimizes potential issues during actual deployment.
Error Handling in PowerShell
Implement robust error handling in your scripts to account for potential failures. Use `try-catch` blocks to manage exceptions and provide meaningful messages:
try {
# Your code here
} catch {
Write-Error "An error occurred: $_"
}
This ensures that you can diagnose problems quickly and maintain a clear log of actions and their outcomes.
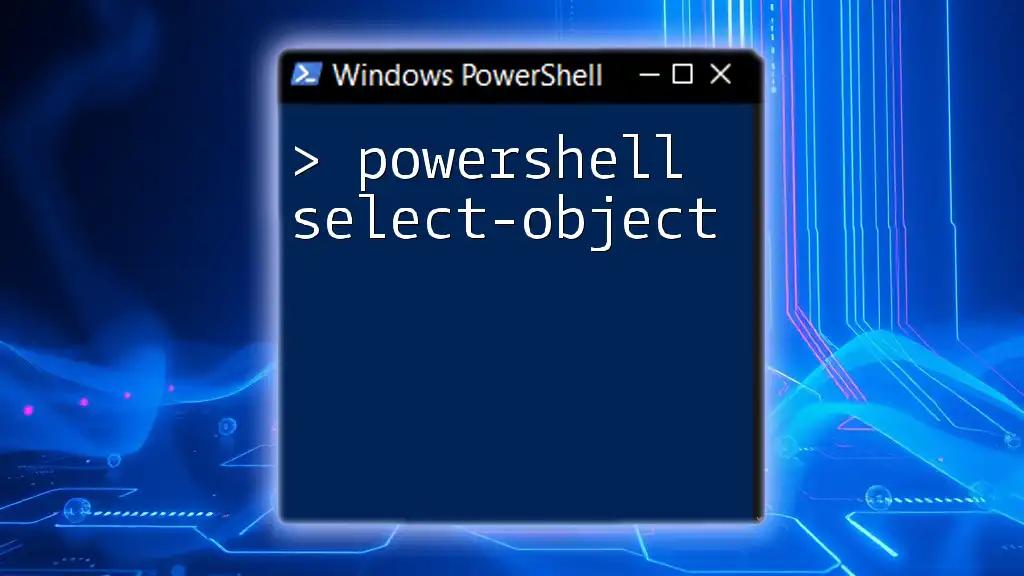
Conclusion
Utilizing SCCM PowerShell detection methods enhances your software deployment strategy, ensuring a seamless experience. By leveraging the different detection types and PowerShell's scripting capabilities, you can automate and streamline your SCCM processes. Don't hesitate to experiment with the examples provided and adapt them to suit your organizational needs.
Taking advantage of these techniques will not only improve your skillset but also significantly benefit your overall SCCM management efforts. Consider exploring further resources to deepen your understanding and keep your knowledge up to date.