When the PowerShell `try-catch` block isn't functioning as expected, it may be due to the fact that the error you're trying to catch isn't terminating the script, which can be resolved by explicitly throwing the error within the `try` block.
try {
# Simulate an error
$nullVariable = $null
$nullVariable.Throw() # This will throw an error
} catch {
Write-Host "An error occurred: $_"
}
Understanding Try Catch in PowerShell
What is a Try Catch Block?
In PowerShell, a Try Catch block is a powerful construct used for error handling. It allows developers to anticipate potential errors in scripts and manage their outcomes gracefully. Without Try Catch, your script might halt execution on encountering an error, which can be frustrating, especially in automated tasks.
Basic Syntax
The general structure of a Try Catch block is straightforward and comprises three primary components:
try {
# Code that might cause an error
} catch {
# Code to handle the error
}
In the `try` block, you place the code that may throw an error. If an error occurs, execution transfers to the `catch` block, where you can define actions to handle the error.
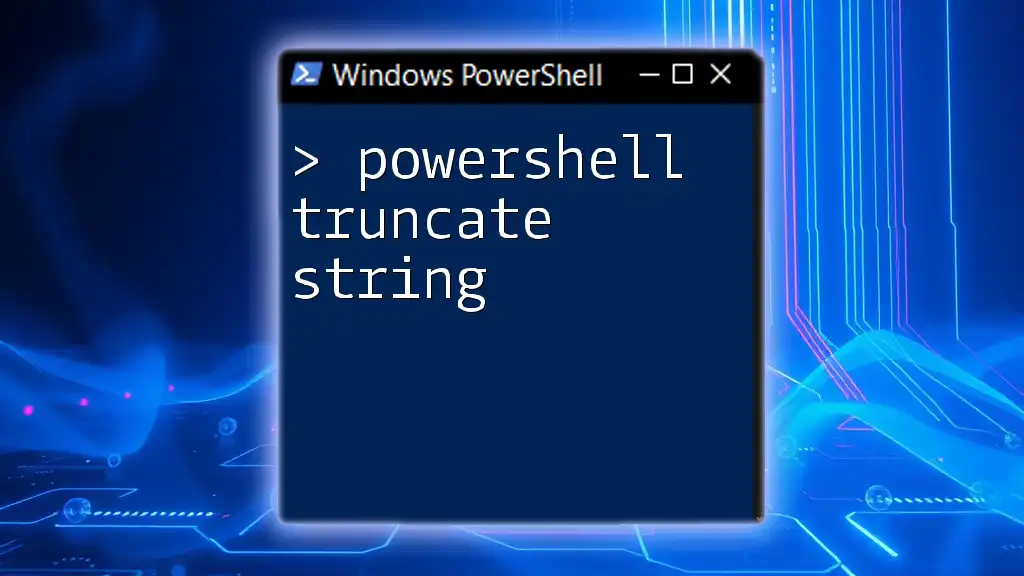
Common Reasons Why Try Catch May Not Work
Errors Outside Try Block
One common pitfall is when errors occur outside of the Try block. Such errors will be unnoticed by the Try Catch mechanism. For instance, consider the following script:
# Error occurs before Try
Write-Host "Script Started"
Get-Content "nonexistentfile.txt" # This will throw an error
try {
Write-Host "This is the try block"
} catch {
Write-Host "Caught an error"
}
In this example, the `Get-Content` command executes before the `try` block and causes an immediate error. As a result, the `catch` block will not be triggered, demonstrating that only errors within the `try` scope are captured.
Non-Terminating Errors
Another reason the Try Catch may seem ineffective is due to non-terminating errors. PowerShell categorizes errors into two types: terminating and non-terminating. A non-terminating error allows the script to continue execution, meaning the Try Catch block will not catch it.
For example:
try {
Write-Host "Attempting to get a service state"
Get-Service -Name "InvalidServiceName" # Non-terminal error
} catch {
Write-Host "Caught an error"
}
Even though the service retrieval fails, your script continues because it is a non-terminating error. Thus, the catch block will not run, leading to confusion regarding PowerShell Try Catch not working.
Catch Block Placement
The placement of your Catch block is crucial for its functionality. One common mistake is placing `catch` statements incorrectly or in a manner that prevents them from executing even when an error arises. Always ensure your `catch` block is directly related to the preceding `try` block or is structured correctly.
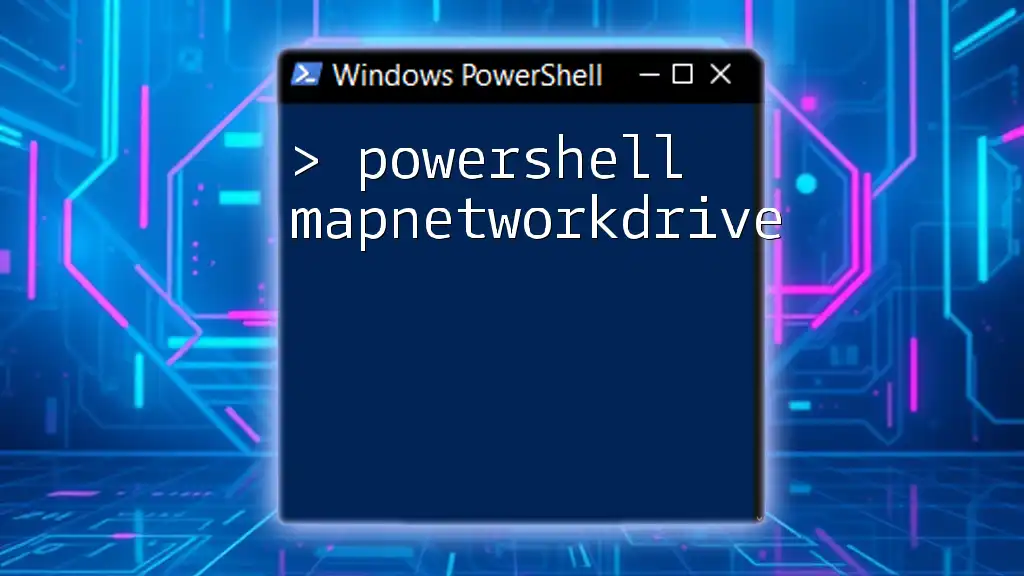
Troubleshooting Techniques
Using Write-Error to Identify Problems
When troubleshooting scripts, incorporating `Write-Error` statements can considerably help in pinpointing errors. By writing custom error messages, you can better diagnose the source of the problem. Consider the following example:
try {
Get-Content "somefile.txt"
} catch {
Write-Error "Error occurred while trying to read the file."
}
If an error occurs, the message "Error occurred while trying to read the file." will be displayed in the error log for easier tracking.
Enabling Error View
Adjusting the `$ErrorActionPreference` variable is another method for improving error handling. By setting this preference to `Stop`, you can convert non-terminating errors into terminating ones, allowing them to be caught by your Try Catch block.
$ErrorActionPreference = "Stop"
try {
Get-Content "anothernonexistentfile.txt"
} catch {
Write-Host "Handled by Catch"
}
In this case, `Get-Content` will throw a terminating error due to the configuration, thus successfully allowing the catch block to execute.
Using the `-ErrorAction` Parameter
For more granular control, you can use the `-ErrorAction` parameter with individual commands to dictate how they handle errors. For instance:
try {
Get-Service -Name "AnotherInvalidService" -ErrorAction Stop
} catch {
Write-Host "Caught the service error"
}
In this scenario, using `-ErrorAction Stop` ensures that if the service retrieval fails, control immediately moves to the catch block.
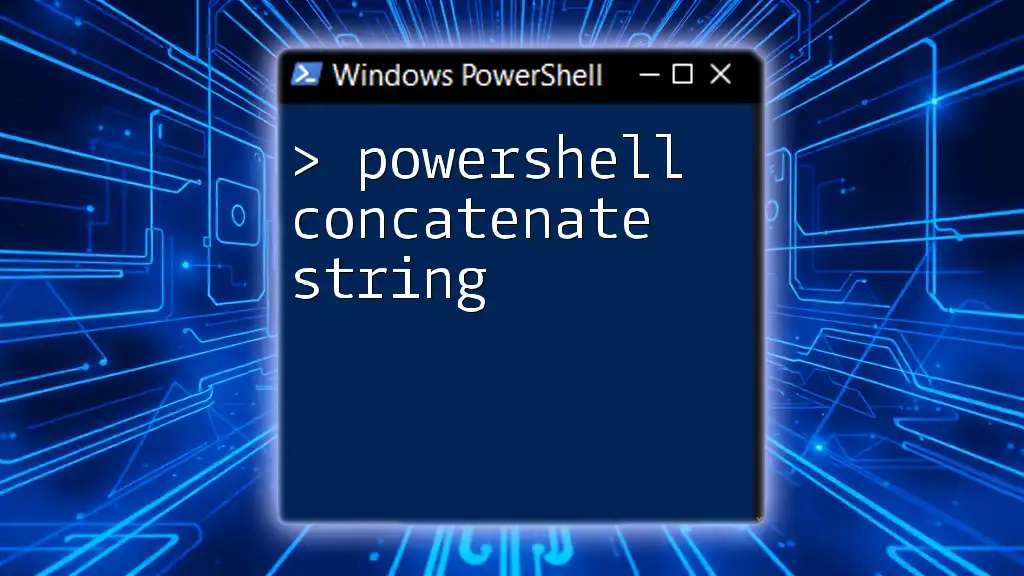
Advanced Try Catch Techniques
Finalizing with Finally Block
PowerShell also supports a `finally` block that executes regardless of whether an error occurs. This feature is useful for cleanup actions or final statements. Here is an example:
try {
# Code that might cause an error
} catch {
# Handle error
} finally {
Write-Host "This block executes regardless of error."
}
The `finally` block guarantees certain code will run, enhancing script stability.
Nested Try Catch Blocks
In some complex scenarios, you may require nested Try Catch blocks. This allows you to handle different levels of errors independently.
try {
try {
# Inner try block
} catch {
# Inner catch block
}
} catch {
# Outer catch block
}
By using this structure, you can manage multiple layers of error handling, which is especially useful in intricate scripts.
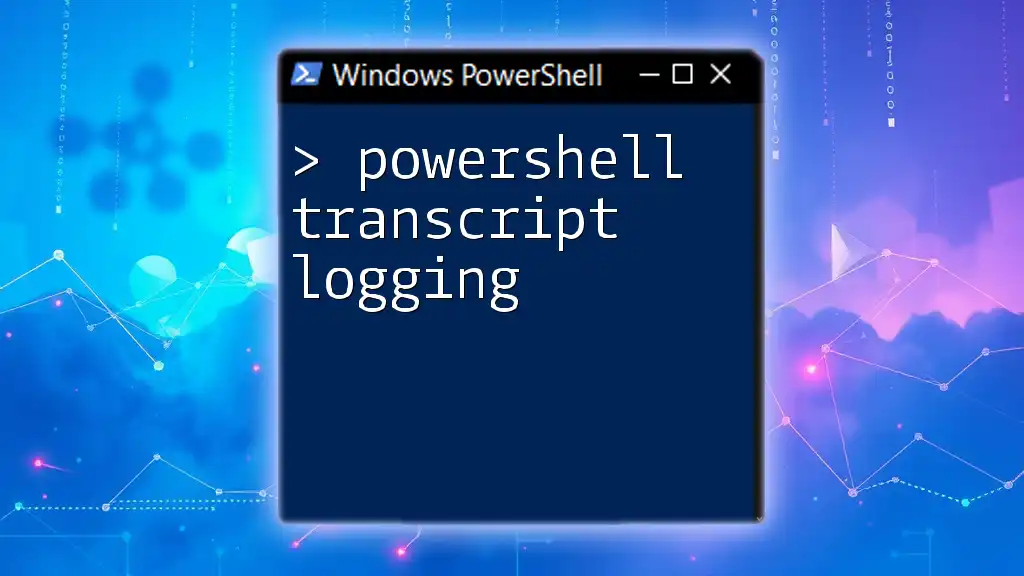
Conclusion
The PowerShell Try Catch not working issue often arises from misunderstandings about error types, block placement, and configuration settings. By being mindful of where errors are processed and incorporating effective troubleshooting techniques, you can significantly improve your error handling capabilities in PowerShell.
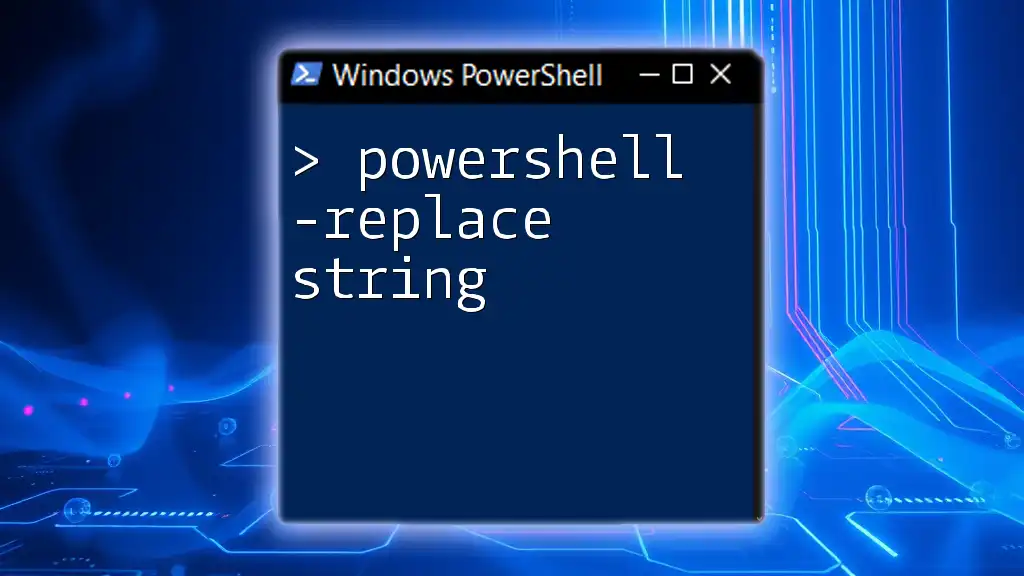
Resources for Further Learning
For those looking to deepen their understanding of PowerShell error handling, consider exploring additional documentation, tutorials, and community forums. Books and online courses can also provide substantial insights into advanced scripting techniques.