In PowerShell, the "not equal" operator (`-ne`) is used to evaluate whether two values are not the same, which can be essential in conditional statements to control the flow of execution based on inequality.
Here’s a simple example:
if ($a -ne $b) {
Write-Host 'The values are not equal.'
}
Understanding Comparison Operators in PowerShell
PowerShell offers a variety of comparison operators that allow you to compare values within your scripts. These operators are crucial for making decisions based on variable values, which forms the backbone of automation tasks and logic flow in your scripts.
Common Comparison Operators
Some of the most frequently used comparison operators in PowerShell include:
- `-eq`: Equals
- `-ne`: Not equal
- `-lt`: Less than
- `-gt`: Greater than
- `-le`: Less than or equal to
- `-ge`: Greater than or equal to
Focus on 'Not Equal' Operator
The `-ne` (not equal) operator is particularly important when you want to evaluate whether two values are not the same. It's differentiated from the `!=` operator, although both perform the same function. Understanding the nuances of `-ne` ensures that your condition checks are efficient, which is critical in script performance.
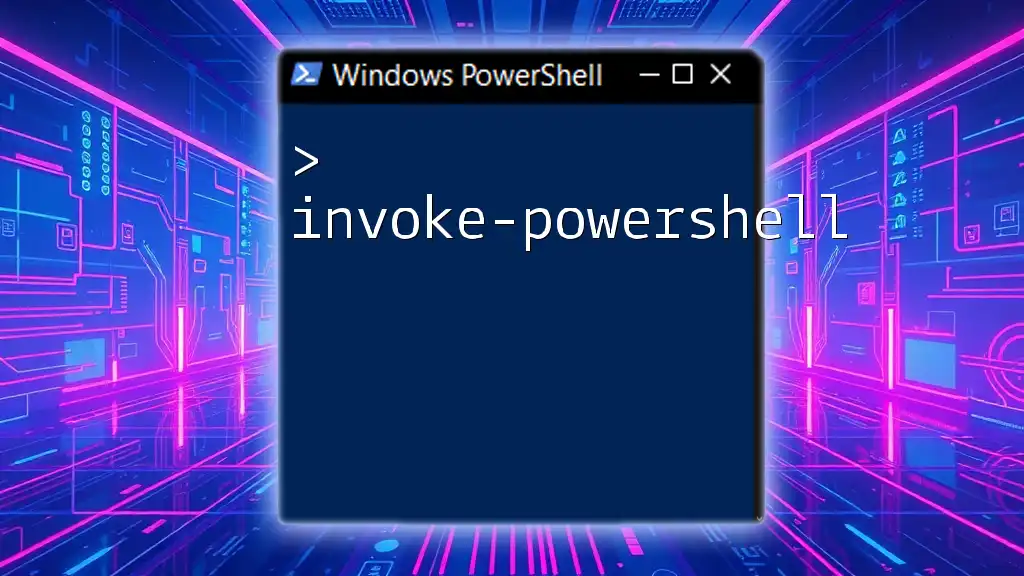
Syntax and Usage of 'Not Equal' Operator
Basic Syntax
The syntax for using the `-ne` operator within an `if` statement is straightforward. Here’s how it typically appears:
if ($variable1 -ne $variable2) {
# Code to execute if the condition is true
}
This simple structure allows you to execute specific code blocks when two variables do not hold equal values.
Usage in Scripts
The `-ne` operator shines in practical scripting. For instance, if you want to prompt a user for input and verify whether the input is different from a specific value, you could write:
$userInput = Read-Host "Enter a number"
if ($userInput -ne 5) {
Write-Output "Your input is not equal to 5."
}
In this scenario, if the user enters a number different from 5, the script will provide straightforward feedback. This immediate validation enhances user experience and script functionality.
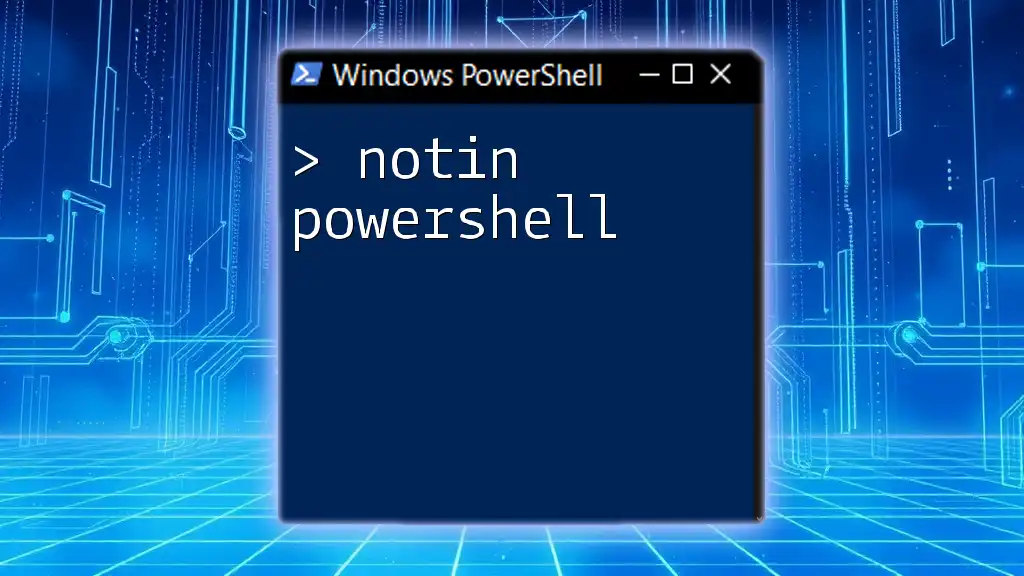
Implementing 'Not Equal' in Conditional Logic
Using `If` Statements with `-ne`
The most fundamental way to implement the `-ne` operator is within an `if` statement. Here’s a basic example:
$item = "apple"
if ($item -ne "orange") {
Write-Output "It's not an orange!"
}
In this context, if the `$item` is not equal to "orange", the condition evaluates to true, and the message is displayed. This simple logic can be extended to many different applications, making it incredibly versatile.
Nesting 'If' Statements
In more complex scenarios, you may find the need to nest your `if` statements. This allows you to check multiple conditions in a more organized way:
$fruit = "banana"
if ($fruit -ne "apple") {
if ($fruit -ne "orange") {
Write-Output "It's neither an apple nor an orange."
}
}
In this example, the script first checks if `$fruit` is not "apple". If this condition holds, it proceeds to check if it’s also not "orange". If both conditions are true, the message confirms that the fruit is neither of those options. Nesting provides better clarity and allows for complex logical flows.
Combining with Logical Operators
You can also incorporate logical operators like `-and` and `-or` to create compound conditions. This expands your scripting capabilities considerably:
$color = "blue"
if ($color -ne "red" -and $color -ne "green") {
Write-Output "The color is neither red nor green."
}
In this example, the condition will only evaluate to true if the `$color` is neither "red" nor "green". Such logical combinations enable you to write powerful, concise scripts to handle various scenarios.
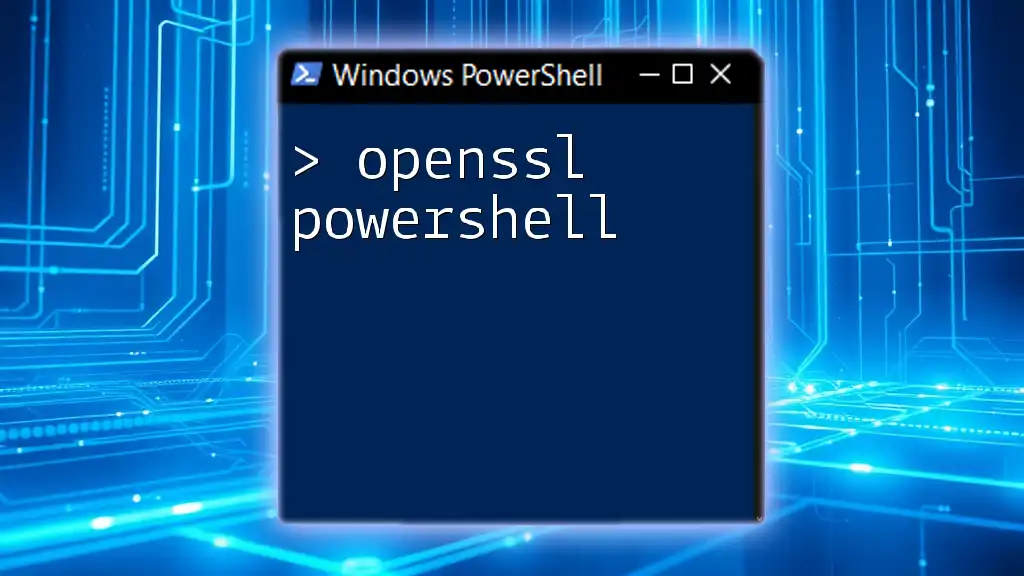
Practical Applications of 'Not Equal' in PowerShell
Common Scenarios
The `-ne` operator proves particularly useful in validating user inputs in scripts. For instance, when creating an application where the user must provide certain values, employing the `-ne` operator can prevent errors or unwanted actions based on incorrect input.
Data Filtering
You can also utilize `-ne` to filter data effectively, useful when working with arrays or collections. For example:
$array = @(1, 2, 3, 4, 5)
$filteredArray = $array | Where-Object { $_ -ne 3 }
In this snippet, the script filters the original array and removes any occurrences of the value 3. Using `-ne` in combination with `Where-Object` allows for flexible data manipulation, which is a common requirement in automation tasks.
Error Handling
Robust scripts often require some form of error handling. The `-ne` operator is invaluable in such cases. It can direct your script to execute alternative actions when a specific condition is not met, guiding users or log responses effectively.
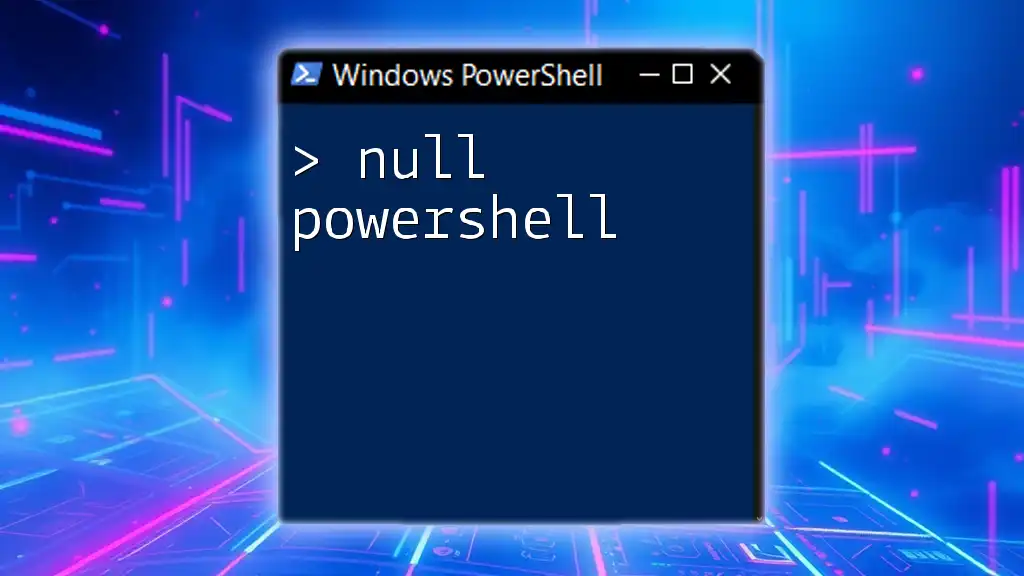
Troubleshooting Common Issues
Common Mistakes
When utilizing the `-ne` operator, errors often arise from typos or misunderstanding its function. One common mistake is assuming that `-ne` compares objects by value without considering their types.
Tips for Debugging
Debugging scripts that include `-ne` can often be simplified by printing out the values being compared first. If a condition isn’t working as expected, checking the values can quickly provide insight. Use `Write-Host` or `Write-Output` to display variable states for troubleshooting.
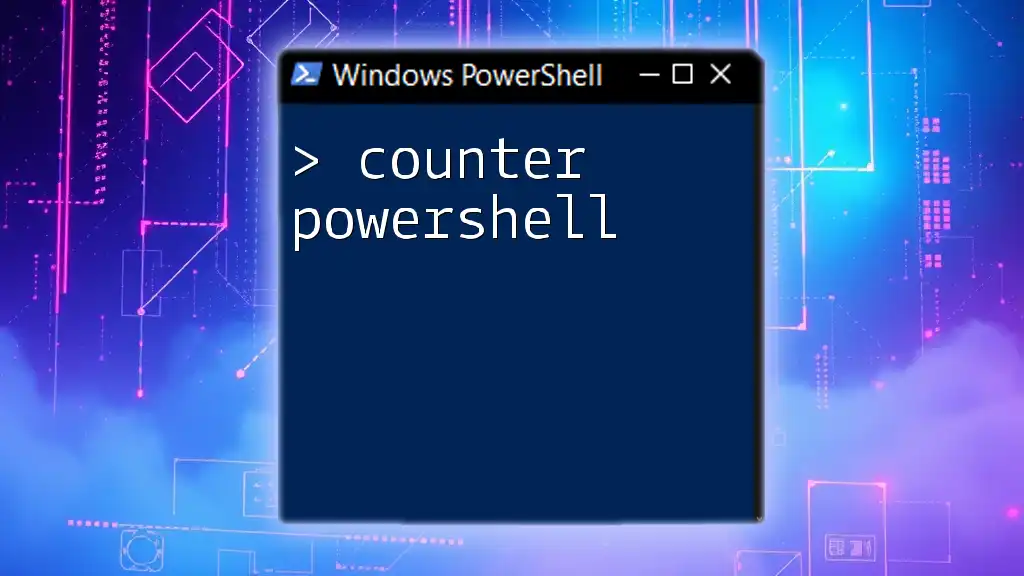
Conclusion
The 'Not Equal' concept in PowerShell is fundamental for any effective scripting practice. Mastering the `-ne` operator will enable you to write more efficient, complex scripts that cater to a variety of logical conditions. Remember, practice is key; experiment with `-ne` in your scripts to fully grasp its capabilities and enhance your PowerShell proficiency.
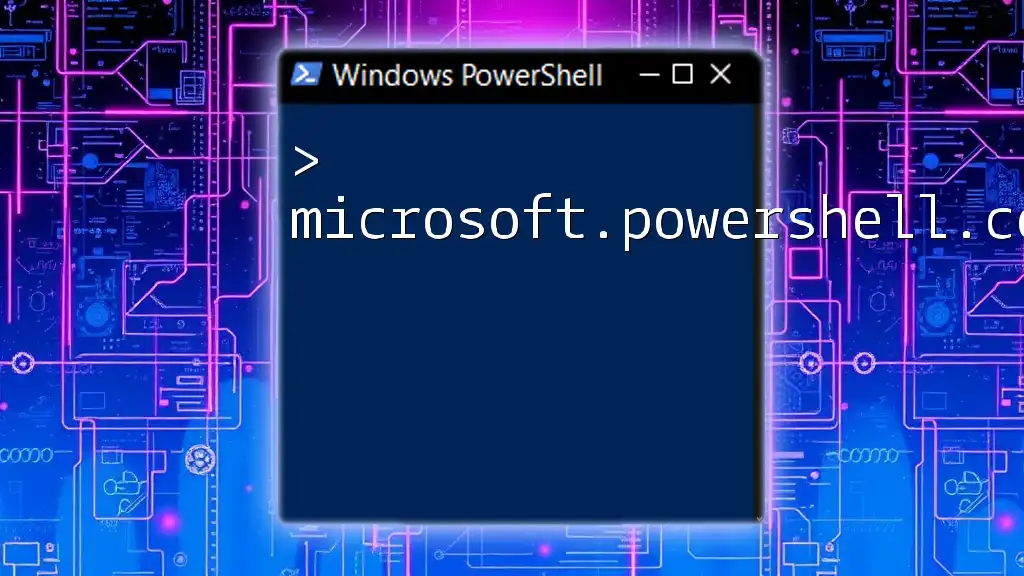
Additional Resources
For further learning, consider checking out official PowerShell documentation, enrolling in online courses, and participating in community forums that focus on PowerShell scripting. These resources can provide the knowledge and support needed to excel in your PowerShell journey.