To perform a silent installation of an MSI package using PowerShell, you can utilize the `msiexec` command with the appropriate flags to suppress user prompts.
msiexec /i "C:\Path\To\YourInstaller.msi" /quiet /norestart
Understanding MSI Files
What are MSI Files?
MSI files, or Microsoft Installer files, are a standard format for installing software on Windows operating systems. They contain all the necessary information and resources needed to install an application, including components, registry entries, and installation settings. Unlike other installation formats, such as EXE files, MSI files are specifically designed to work with Windows Installer, making them more suitable for enterprise deployment scenarios.
Why Use Silent Installation?
Silent installation refers to the process of installing software without any user interaction or graphical user interface (GUI). The benefits of this method are substantial, especially in enterprise environments where deploying software to multiple machines quickly and seamlessly is crucial.
- Automation: Silent installs can be scripted and run automatically, reducing the need for manual input.
- User Experience: Users can continue working without interruption, as they are not faced with installation dialogs.
- Efficiency: System administrators can deploy applications across multiple machines simultaneously.
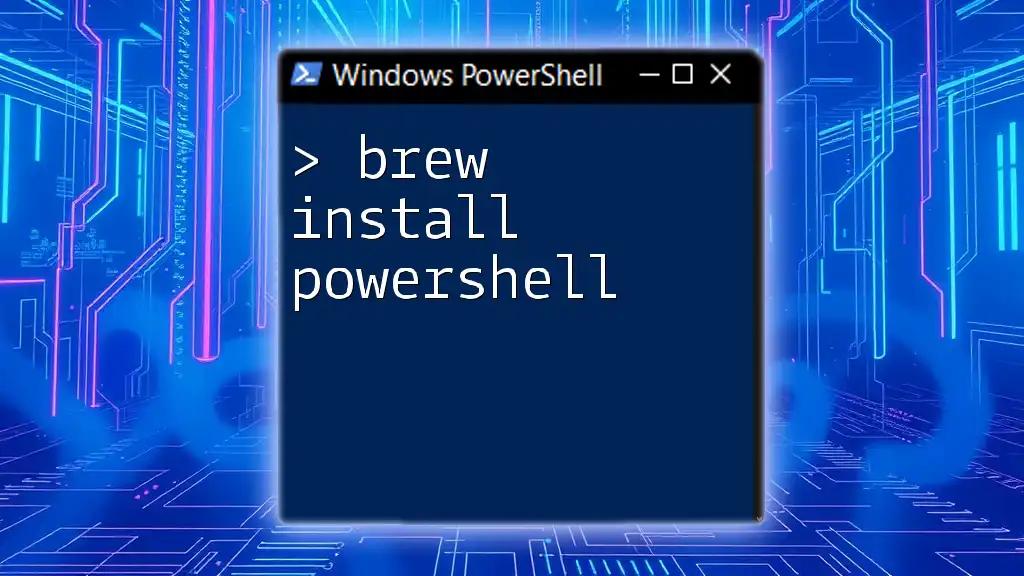
PowerShell and MSI Installation
Introduction to PowerShell
PowerShell is a powerful scripting language developed by Microsoft, primarily used for task automation and configuration management. With its robust command-line scripting capabilities, PowerShell provides an efficient way to manage Windows-based systems and automate repetitive administrative tasks. Its compatibility with various Windows components, including MSI installers, makes it an invaluable tool for software deployment.
How to Install MSI Files Using PowerShell
Basic Command Structure
To perform a silent install of an MSI file using PowerShell, you will commonly use the `msiexec.exe` utility. The command structure for installing an MSI silently is straightforward. Here’s a basic example:
msiexec.exe /i "C:\Path\To\YourInstaller.msi" /qn
In this command:
- /i signals the installer to install the MSI file specified.
- /qn denotes a "quiet" installation with no user interface.
Breakdown of Command Parameters
Understanding the command parameters is vital for successful installation. The /i parameter is utilized to initiate the installation process, while /qn is crucial for ensuring the process runs without any GUI prompts. This can greatly streamline the installation experience, particularly in environments requiring zero-touch deployments.
You can also explore additional options like /qb (basic user interface) or /l*v (to enable logging with verbose output) for more control over the installation process.
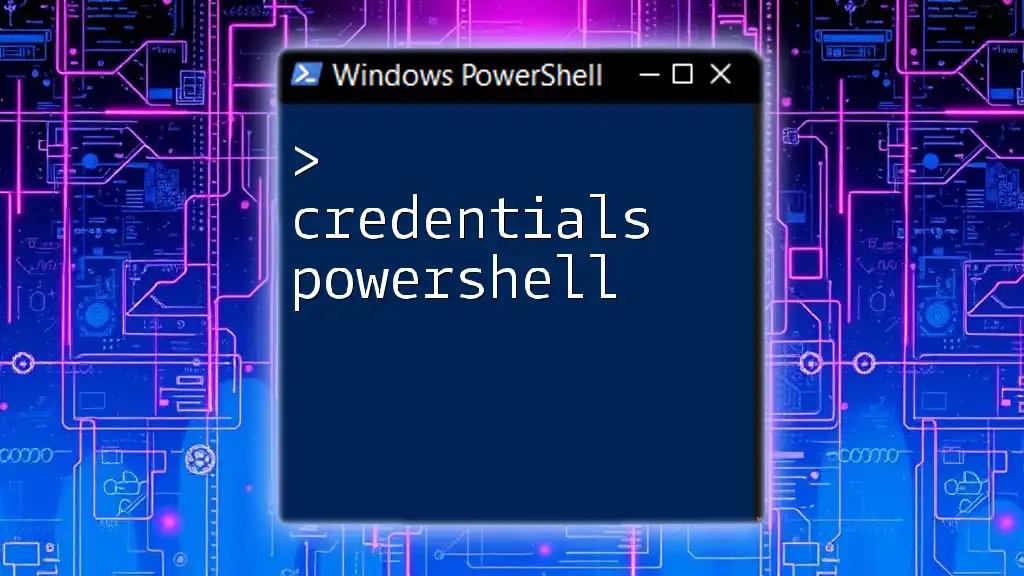
Performing Silent Installation via PowerShell
Setting Up Your Environment
To execute silent installations effectively, it’s essential to prepare your PowerShell environment. Ensure that Windows PowerShell is installed and updated to the latest version. Additionally, be mindful of the execution policy. For tasks involving scripts, it is recommended to set this Policy to allow running scripts by implementing the following command:
Set-ExecutionPolicy RemoteSigned -Scope CurrentUser
Executing a Silent MSI Install
Example: Silent Installation Command
Now that your environment is ready, you can proceed with executing the silent install. Here’s a complete example script that both installs the MSI and waits for the process to complete:
$msiPath = "C:\Path\To\YourInstaller.msi"
Start-Process msiexec.exe -ArgumentList "/i `"$msiPath`" /qn" -Wait
This script sets the path to your MSI file and uses `Start-Process` to initiate the installation silently. The `-Wait` parameter ensures that PowerShell waits for the installation process to complete before continuing.
Verifying Installation
After the installation has concluded, it’s important to verify whether it was successful. You can do this by querying the installed software list. Here’s an example command to check if the software installed successfully:
Get-WmiObject -Class Win32_Product | Where-Object { $_.Name -like "YourSoftwareName*" }
This command retrieves installed software and filters the results based on the product name you specify.
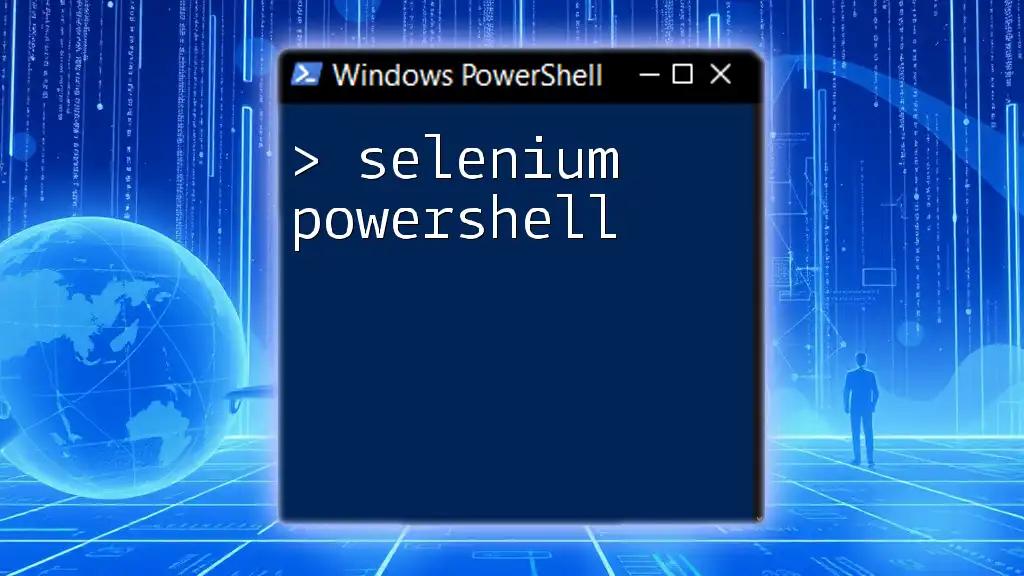
Advanced Silent Installation Techniques
Installing Multiple MSI Packages
In many cases, you might need to install several MSI packages at once. The following example demonstrates how to iterate through multiple MSI files and install them silently:
$msiList = @("C:\Path\To\First.msi", "C:\Path\To\Second.msi")
foreach ($msi in $msiList) {
Start-Process msiexec.exe -ArgumentList "/i `"$msi`" /qn" -Wait
}
This script creates an array of MSI file paths and executes a silent install for each one in the array.
Error Handling in Silent Installations
Common Installation Errors
Even with careful planning, errors can occur during the installation process. Common MSI-related errors may include:
- Access Denied: Occurs when proper permissions are not set.
- File not found: When the specified MSI path is incorrect or the file is missing.
Example of Adding Error Handling
To enhance the installation script with error handling, you can add checks to examine the exit code after running the installation. Here’s how to add error handling to your script:
$process = Start-Process msiexec.exe -ArgumentList "/i `"$msiPath`" /qn" -Wait -PassThru
if ($process.ExitCode -ne 0) {
Write-Host "Installation failed with exit code: $($process.ExitCode)"
} else {
Write-Host "Installation succeeded!"
}
This script captures the exit code of the `msiexec` process and provides feedback based on whether the installation was successful or not.
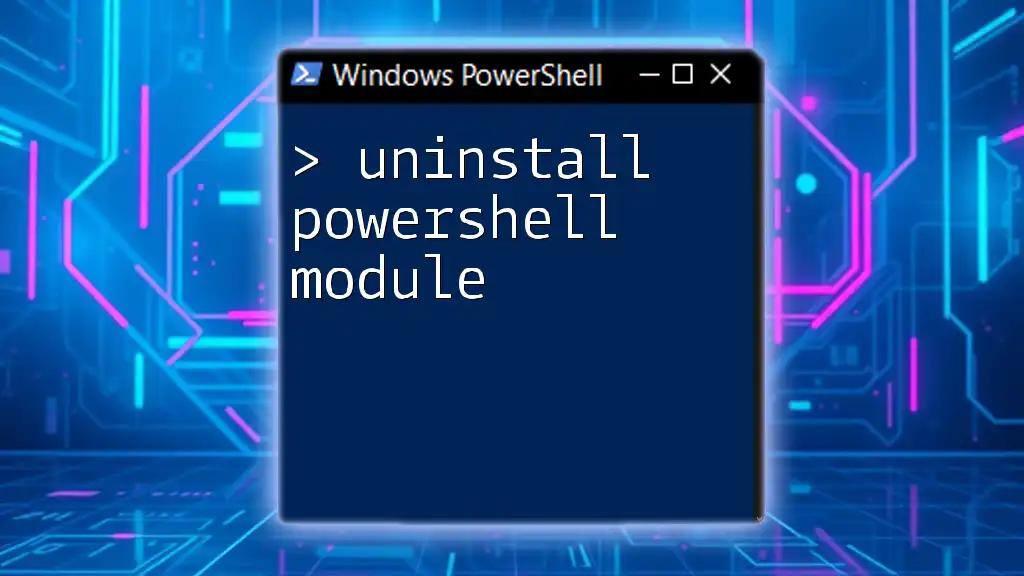
Conclusion
In this guide, we explored the use of PowerShell for performing silent installations of MSI files. By mastering these techniques, you will enhance your software deployment strategies and streamline the installation process within your organization. Embracing PowerShell commands enables system administrators to work efficiently in managing their software landscape, making it an indispensable skill in today's IT environment.
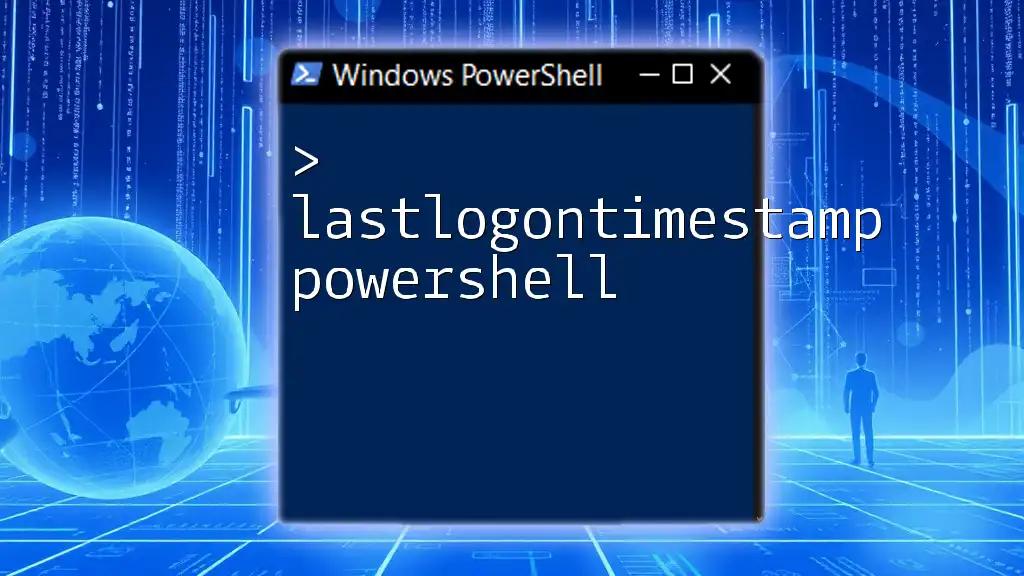
Additional Resources
For further learning, consider exploring the following resources:
- Official Microsoft documentation on PowerShell and MSI.
- Tutorials focused on PowerShell scripting for automation and administrative tasks.